차트의 scale을 정했다면 axis로 그려 차트를 보기 편하게 하자.
<script src="https://cdn.jsdelivr.net/npm/d3@7"></script>
<div id="chart"></div>
<script>
const margin = {top: 20, right: 30, bottom: 30, left: 40};
const width = 960 - margin.left - margin.right;
const height = 960 - margin.top - margin.bottom;
// Create the SVG container and set the origin.
const svg = d3.select("#chart").append("svg")
.attr("width", width + margin.left + margin.right)
.attr("height", height + margin.top + margin.bottom)
// linear
const x = d3.scaleLinear().domain([0, 100]).range([0, 500]);
</script>
선형 scale로 위와 같이 코드를 짠다. 이제 축을 원하는 방향에 그리면 된다.
axisTop(scale)
축을 아래에 그리고 스케일을 축 위에 그린다.
const gx = svg.append("g")
.attr("transform", `translate(10,${height - margin.bottom})`)
.call(d3.axisTop(x));
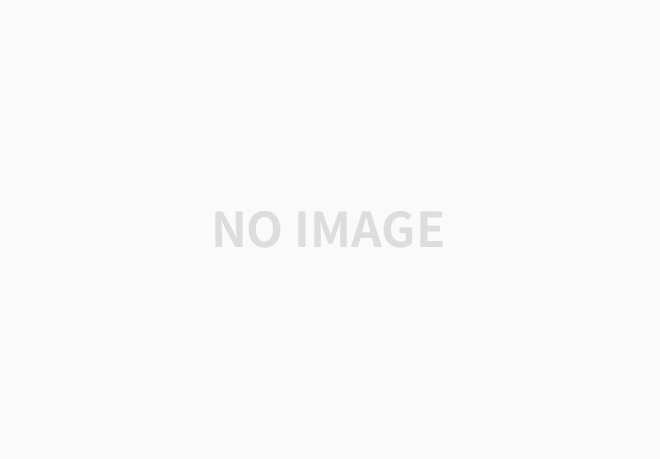
axisRight(scale)
축을 왼쪽에 그리고 스케일을 축 오른쪽에 그린다.
const gx = svg.append("g")
.attr("transform", `translate(10,${margin.bottom})`)
.call(d3.axisRight(x));
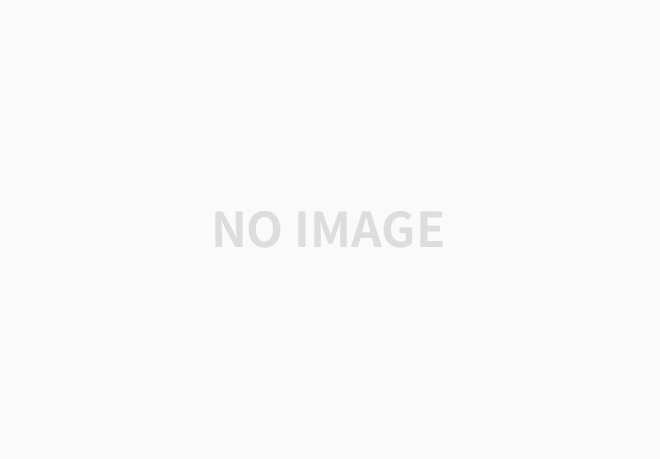
axisLeft(scale)
축을 왼쪽에 그리고 스케일을 축 왼쪽에 그린다.
const gx = svg.append("g")
.attr("transform", `translate(30,${margin.bottom})`)
.call(d3.axisLeft(x));
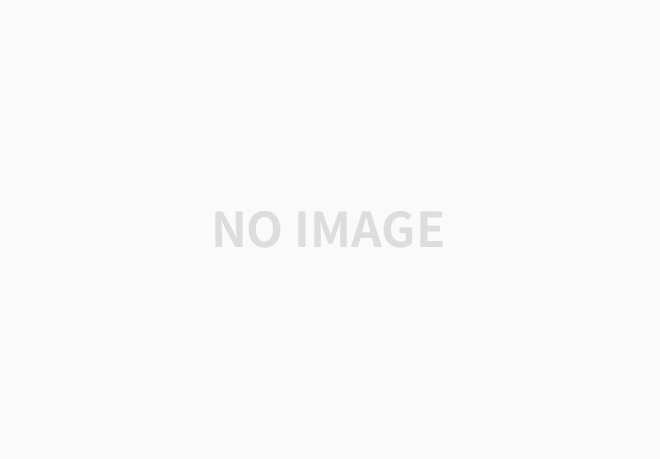
axisBottom(scale)
축을 아래에 그리고 스케일을 축 아래에 그린다.
const gx = svg.append("g")
.attr("transform", `translate(10,${height - margin.bottom})`)
.call(d3.axisBottom(x));

Ticks
틱은 축에 그려진 값들과 간격을 조절하는데 유용하다.
const axis = d3.axisBottom(x);
axis.ticks(10, ",.02f"); // tick 개수
axis.tickFormat(d3.format(",.02f")); // tick 포맷
axis.tickValues([1, 2, 3, 5, 8, 13, 21, 50, 100]); // tick 에 특정값만 표시
axis.tickSize(30); // tick 길이
axis.tickPadding(10); // tick과 value사이 공간 default 3
const gx = svg.append("g")
.attr("transform", `translate(10,${height - margin.bottom})`)
.call(axis);
우선, 틱의 개수를 정할 수 있다. 그리고 포맷 또한 입힐 수 있다.
axis.ticks(10, ",.02f");
틱 개수를 정하고 나중에 포맷을 아래와 같이 입힐 수도 있다.
axis.tickFormat(d3.format(",.02f"));

틱 값을 특정한 값만 표시되게 하려면 다음과 같이 하면 된다.
axis.tickValues([1, 2, 3, 5, 8, 13, 21, 50, 100]);

그리고 아래와 같이 하면 틱 길이가 조절된다.
axis.tickSize(30);
틱과 값 사이의 공간을 좁히거나 늘리고 싶으면 아래와 같이 하면 된다.
axis.tickPadding(10);
지금까지 axis에 대해 필수적인 부분을 알아보았다. 이제 데이터만 다룰 수 있다면 기본적인 차트를 그릴 준비는 끝났다.
'Programming > D3.js' 카테고리의 다른 글
[01. D3.js] 004. D3-Selection (0) | 2025.02.06 |
---|---|
[01. D3.js] 002. D3-Scale (0) | 2024.07.17 |
[01. D3.js] 001. D3.js란 (0) | 2024.07.14 |